Dos and Don'ts
Importing classes
For performance reasons, FirstSpirit or Java classes should always be imported into the header of a script.
Example:
//!Beanshell
// bad
if (myVar instanceof java.lang.String) {
print("its a string!");
}
//!Beanshell
// Good!
import java.lang.String;
if (myVar instanceof String) {
print("its a string!");
}
When scripting with BeanShell there are a few rules which a template developer should adhere to, to design low-maintenance and efficient scripts.
Blocking objects (locking)
The setting of blocks (locks) should always be carried out according to the following schema:
//!Beanshell
import de.espirit.firstspirit.access.store.LockException;
try {
elm.setLock(true, false);
try {
...
elm.save("some comment", false);
} catch (Exception e) {
// catch handling
} finally {
elm.setLock(false, false);
}
} catch (LockException e) {
// Element locked, catch handling
}
This is the only way to ensure safe and reliable removal of the lock.
Iteration via the children of an element
It frequently occurs that a user wants to iterate across all children of a specific element (e.g. folder).
Here it is important to note that the list (especially if all children are considered recursively) can be very long and therefore you should always work with iterators (never with arrays or complete lists).
Here is code example for correct iteration across the children of an element in the tree, whereby the children are filtered according to a specific class (here: “MyClass”).
//!Beanshell
// ..Determination of the variable folder...
for (elem : folder.getChildren(MyClass.class, true).iterator()) {
print(elm.getSomeValue(...));
}
Log outputs
The output of a printout or a variable takes place in BeanShell via the print command: print():
//!Beanshell
// bad
print()
This output should be replaced by differentiated output using the three logging methods:
- logInfo
- logError
- logDebug
The log methods are available in the respective script context.
Example:
//!Beanshell
// good
context.logDebug(...)
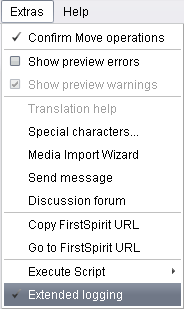
Extras – Activate Extended Logging
The advantage is the logging of scripts can be easily influenced. For example, “extended logging” can be activated for scripts in the FirstSpirit SiteArchitect (see Figure or Extras (→Documentation FirstSpirit SiteArchitect)).
Certain information is displayed or output in the “extended logging” only and can be used for debugging a script. If that is no longer wanted the logging can be simply deactivated again.