Startseite / Plugin-Entwicklung / SiteArchitect-Erweiterungen / Interaktive Features / Kontextmenüeinträge / Codebeispiel
Context Menu Items: Code Example
This example illustrates the code structure to implement context menu items which will be shown in either the New or Plug-ins submenus of store element context menus.
A context menu items plug-in consists of a two-tier class structure:
The first tier defines the plug-in itself and determines which second-tier objects (see below) should be offered as context menu items, depending on the current operational context. The interface that is implemented determines in which submenu the context menu items will be displayed.
- "New" Context Menu Items Plug-In
serves as a container for and provider of one or more individual plug-in items that represent individual context menu items. These items will be displayed in the New submenu of store element context menus. - "Plug-Ins" Context Menu Items Plug-In
serves as a container for and provider of one or more individual plug-in items that represent individual context menu items. These items will be displayed in the Plug-Ins submenu of store element context menus.
The second tier defines individual plug-in items; each item will be represented by its own context menu entry, may define its own visibility context (based on store elements or other criteria) and provides its own, specific action.
- Executable Context Menu Item
provides a button definition including title and icon, and defines an executable action that will be executed on the server side and handle interaction with users - Grouping Context Menu Item
provides a button definition including title and icon, and defines an executable action that will be executed on the server side and handle interaction with users; this button may also specify one or context menu item objects which will be rendered in a drop-down list alongside the toolbar button - Separator Context Menu Item
causes a separator graphic to be drawn in its place; this item has neither executable action nor configurable representation
![]() |
A context menu items plug-in may contain one or more of the second-tier item classes. Each item will be represented by an individual context menu entry, provide its own actions and define its own availability based on criteria such as context store element, etc. However, each of the second-tier button items returned by getItems() should return a unique combination of label and icon graphic such that individual context menu items remain distinguishable. |
The "New" Context Menu Items Plug-In
The New context menu items plug-in implements the interface JavaClientContextNewMenuItemsPlugin and is responsible for collecting and returning a list of context menu items that will be shown in the New submenu of store element context menus. This class on its own has no representation in ContentCreator's user interface.
public class ExampleJavaClientContextNewMenuItemsPlugin implements JavaClientContextNewMenuItemsPlugin {
// Field definitions for objects that are required during the lifetime of a class instance.
private BaseContext _context;
@Override
public void setUp(@NotNull final BaseContext context) {
_context = context;
}
@Override
public void tearDown() {
}
@NotNull
@Override
public Collection<? extends JavaClientToolbarItem> getItems() {
// Create and return an array list that includes individual context menu item objects.
return Arrays.asList(
new ExampleExecutableContextMenuItem(),
SeparatorContextMenuItem.INSTANCE,
new ExampleGroupingContextMenuItem()
);
}
/* Include one or several private static classes here that implement context menu item interfaces,
either ExecutableContextMenuItem or GroupingContextMenuItem. */
}
void setUp()
This method is called by the SiteArchitect to set up the plug-in prior to polling it for available context menu items.
void tearDown()
This method is called by the SiteArchitect as the context menu items plug-in is about to be destroyed.
Collection<? extends JavaClientContextMenuItem> getItems()
After instantiating and setting up the context menu items plug-in object, SiteArchitect will call getItems() to poll for a list of available, individual context menu items. This method should return all available items defined as nested classes within the plug-in class; SiteArchitect will autonomously call the appropriate, item-specific methods of each item returned by getItems() to determine in which context(s) each item should be shown and marked as active.
The "Plug-ins" Context Menu Items Plug-In
The Plug-ins context menu items plug-in implements the interface JavaClientContextPluginsMenuItemsPlugin and is responsible for collecting and returning a list of context menu items that will be shown in the Plug-ins submenu of store element context menus. This class on its own has no representation in ContentCreator's user interface.
public class ExampleJavaClientContextPluginsMenuItemsPlugin implements JavaClientContextPluginsMenuItemsPlugin {
// Field definitions for objects that are required during the lifetime of a class instance.
private BaseContext _context;
@Override
public void setUp(@NotNull final BaseContext context) {
_context = context;
}
@Override
public void tearDown() {
}
@NotNull
@Override
public Collection<? extends JavaClientToolbarItem> getItems() {
// Create and return an array list that includes individual context menu item objects.
return Arrays.asList(
new ExampleExecutableContextMenuItem(),
SeparatorContextMenuItem.INSTANCE,
new ExampleGroupingContextMenuItem()
);
}
/* Include one or several private static classes here that implement context menu item interfaces,
either ExecutableContextMenuItem or GroupingContextMenuItem. */
}
void setUp()
This method is called by the SiteArchitect to set up the plug-in prior to polling it for available context menu items.
void tearDown()
This method is called by the SiteArchitect as the context menu items plug-in is about to be destroyed.
Collection<? extends JavaClientContextMenuItem> getItems()
After instantiating and setting up the context menu items plug-in object, SiteArchitect will call getItems() to poll for a list of available, individual context menu items. This method should return all available items defined as nested classes within the plug-in class; SiteArchitect will autonomously call the appropriate, item-specific methods of each item returned by getItems() to determine in which context(s) each item should be shown and marked as active.
Executable Context Menu Item
The following code implements an executable context menu item based on the interface ExecutableContextMenuItem and an action that is able to use the full FirstSpirit APIs to produce functionality.
In this example, it is a private class nested within its parent, context menu items plug-in class.
![]() |
Since any store element context menu instance can be associated with more than one store element (due to multiple selection), all context menu item methods receive a context object of type ContextMenuContext. As opposed to most other context objects which relate to single store elements per instance, the ContextMenuContext class features a getElements() method which returns a Listable of all IDProvider objects selected at the time the context menu instance was created. |
private static class ExampleExecutableContextMenuItem implements ExecutableContextMenuItem {
ExampleExecutableContextMenuItem() {
}
public String getLabel(@NotNull final ContextMenuContext context) {
return "Example Executable Context Menu Item";
}
public Icon getIcon(@NotNull final ContextMenuContext context) {
return null;
}
// Ensure the context menu was called on only one store element; don't show this item for template store elements.
public boolean isVisible(@NotNull final ContextMenuContext context) {
final List<IDProvider> elementList = context.getElements().toList();
if (elementList.size() == 1) {
final IDProvider element = elementList.get(0);
return !(
element instanceof Template ||
element instanceof TemplateFolder ||
element instanceof TemplateStoreRoot
);
}
return false;
}
// Ensure the context menu was called on only one store element; disable this item if the element is a store root.
public boolean isEnabled(@NotNull final ContextMenuContext context) {
final List<IDProvider> elementList = context.getElements().toList();
return elementList.size() == 1 && !(elementList.get(0) instanceof Store);
}
public void execute(@NotNull final ContextMenuContext context) {
final OperationAgent operationAgent = context.requireSpecialist(OperationAgent.TYPE);
final RequestOperation requestOperation = operationAgent.getOperation(RequestOperation.TYPE);
requestOperation.setKind(RequestOperation.Kind.INFO);
requestOperation.perform("This message box is generated by the Example Executable Toolbar Item.");
}
}
String getLabel()
This method is responsible for providing the item's display name that will be shown in its context menu entry representation.
Icon getIcon()
This method may provide an Icon graphic that will be shown alongside the context menu item's label. If this method returns a null value, the context menu item will be shown with its label text only.
boolean isVisible()
This method is responsible for determining in which context the menu item is to be displayed. If the item is to be displayed, the boolean value true should be returned; the value false will suppress display of this item.
boolean isEnabled()
The method isEnabled() indicates if the item's menu entry should be active in a given context. If it is to be active, this method should return the boolean value true. If the context menu item is to be inactive, the boolean value false will cause the entry to be displayed with decreased contrast to produce a muted representation; while the item will remain visible (given that isVisible() returns true in the same context), the item's action code will not be executed on-click.
void execute()
This method will be called by the SiteArchitect once a user clicks the item's context menu entry. execute() is treated as a point of entry into executable code to carry out the item's action and is not expected to return any value.
Grouping Context Menu Item
The following code implements a grouping context menu item based on the interface GroupingContextMenuItem which has no own executable action but merely provides one or more context menu item objects that will be grouped together as a submenu.
In this example, it is a private class nested within its parent, context menu items plug-in class.
![]() |
Since any store element context menu instance can be associated with more than one store element (due to multiple selection), all context menu item methods receive a context object of type ContextMenuContext. As opposed to most other context objects which relate to single store elements per instance, the ContextMenuContext class features a getElements() method which returns a Listable of all IDProvider objects selected at the time the context menu instance was created. |
private static class ExampleGroupingContextMenuItem implements GroupingContextMenuItem {
ExampleGroupingContextMenuItem(@NotNull final BaseContext context) {
}
public String getLabel(@NotNull final ContextMenuContext context) {
return "Example Grouping Context Menu Item";
}
public Icon getIcon(@NotNull final ContextMenuContext context) {
return null;
}
// Ensure the context menu was called on only one store element; don't show this item for template store elements.
public boolean isVisible(@NotNull final ContextMenuContext context) {
final List<IDProvider> elementList = context.getElements().toList();
if (elementList.size() == 1) {
final IDProvider element = elementList.get(0);
return !(
element instanceof Template ||
element instanceof TemplateFolder ||
element instanceof TemplateStoreRoot
);
}
return false;
}
// Ensure the context menu was called on only one store element; disable this item if the element is a store root.
public boolean isEnabled(@NotNull final ContextMenuContext context) {
final List<IDProvider> elementList = context.getElements().toList();
return elementList.size() == 1 && !(elementList.get(0) instanceof Store);
}
// Reuse some executable context menu item instances as entries of the submenu.
public List<JavaClientContextMenuItem> getSubItems(@NotNull final ContextMenuContext context) {
return Arrays.asList(
new ExampleExecutableContextMenuItem(),
SeparatorContextMenuItem.INSTANCE,
new ExampleExecutableContextMenuItem()
);
}
}
String getLabel()
This method is responsible for providing the item's display name that will be shown in its context menu entry representation.
Icon getIcon()
This method may provide an Icon graphic that will be shown alongside the context menu item's label. If this method returns a null value, the context menu item will be shown with its label text only.
List<JavaClientContextMenuItem> getSubItems()
This method will be called by SiteArchitect to collect further context menu items which should be displayed in a submenu attached to this grouping context menu item.
The List may contain objects of types ExecutableContextMenuItem and GroupingContextMenuItem as well as SeparatorContextMenuItem instances.
boolean isVisible()
This method is responsible for determining in which context the menu item is to be displayed. If the item is to be displayed, the boolean value true should be returned; the value false will suppress display of this item.
boolean isEnabled()
The method isEnabled() indicates if the item's menu entry should be active in a given context. If it is to be active, this method should return the boolean value true. If the context menu item is to be inactive, the boolean value false will cause the entry to be displayed with decreased contrast to produce a muted representation; while the item will remain visible (given that isVisible() returns true in the same context), the item's action code will not be executed on-click.
Separator Context Menu Item
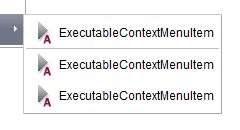
A separator, placed between several context menu items
An object of type SeparatorContextMenuItem may be used to draw a separator in its place in context menu item lists.
This item is an object of type Enum and features no configurable options. When used in item-providing methods such as getItems() or getSubItems(), it should simply be referred to as SeparatorContextMenuItem.INSTANCE.